in this tutorial set we will see how to do Firestore CRUD operations with flutter. we will also see how to create simple queries in Firestore. this will be consist of 3 parts.
Note : All the Working State Code for these tutorials can be found in : https://github.com/SrilalS/Flutter-Tutorial
Step 1
Add firestore to the Flutter Project. to do it please first read the following tutorial.
https://medium.com/@srilalsachintha/getting-started-with-firestore-flutter-android-31bee8c1412c
Step 2
lets first create a firestore reference in our project. there are few ways to do this.
- Document Reference — in this we only refer to a single document in the database. this can be any document in our database as long as you have the path to that document. this will not retrieve the child collections inside the referred Document.
- Collection Reference — here we refer to a whole collection. but similar to the document reference this will not retrieve the child Collections inside the referred Collection. we will discuss this in the next tutorial. link to that will be added after that has been published.
the reason why you will not get the sub Collection is that the queries in Firestore is shallow by default. it only look in the surface.
First, lets explore Document Referencing.
Take a look at my Database Structure first.
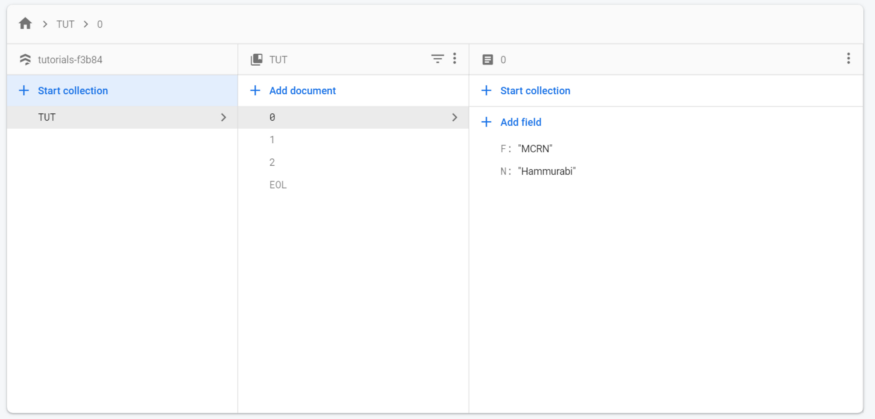
I have a Root Collection named ‘TUT’. inside it i have 4 documents. now i’m gonna refer to the first document in this collection.
create a document reference after the state creation,
final docref = Firestore.instance.collection(‘TUT’).document(‘0’);
note that i’m going through the collection to the specific document. this is what called document reference.
now you can use ‘docref’ to do the CRUD operations. lets retrieve the data first.
in here data retrieved from the Firebase is retrieved as a data-snapshot named ‘doc’. under that we have ‘data’. it’s a String Map Data Structure. you have to go to the correct data via the correct path. doc-snapshot object named ‘doc’.
the ‘then’ operator activates after the data is fully retrieved. what happening here is that after the data is downloaded it assign the data to a doc-snapshot object.
for the sake of keeping things simple i’m assigning the ’N’ to a variable named ‘txt’. i also have ‘F’ in the Document. (Again Refer to the Screenshot of the Database.). let’s assign it to ‘faction’ variable.
faction = doc.data[‘F’];
to Write data you can use ‘SetData’ this is the same thing used to update the document data. if you pass a field that is already there in the db it will be overwritten. if you go with the proper ways you can your ‘update’ instead of ‘SetData’.
You can put Arrays in here. also you can put new data fields via this.
’N’ : ‘Donnager’,
‘F’ : ‘MCRN’,
‘C’ : ‘Dreadnought’
the ‘C’ will be added as a new field.
if the document is missing in the database it will be created. document id is already given when we create the document reference.
To delete the document simply use the following. refer to the document reference and pass delete()
docref.delete();
In the next tutorial we will discuss about collection reference.
Note : All the Working State Code for these tutorials can be found in : https://github.com/SrilalS/Flutter-Tutorial