In this tutorial set we will see how to do Firestore CRUD operations with flutter. we will also see how to create simple queries in Firestore. this will be the 2nd Part of consist Firebase CRUD Operations With Flutter. Read the Previous one from here.
Note : All the Working State Code for these tutorials can be found in : https://github.com/SrilalS/Flutter-Tutorial
We begin this tutorial with 2 Assumptions.
- You have successfully added Firebase to your project (Required)
- You have read the Previous Tutorial (Optional)
To Learn about how to add Firebase to flutter read the following first. (10mins)

as is described in the previous tutorial there are 2 types of Firestore references available.
- Document Reference — in this we only refer to a single document in the database. this can be any document in our database as long as you have the path to that document. this will not retrieve the child collections inside the referred Document.
- Collection Reference — here we refer to a whole collection. but similar to the document reference this will not retrieve the child Collections inside the referred Collection. we will discuss this in this tutorial.
Collections what holds documents. Collection can have many documents inside. these documents doesn’t have to have a same structure.
A Single Document Can Have Many Collections. and those Collections can have many documents in them.
So a Firestore Database has a architecture similar to Tree-Like Architecture but with Some Key Differences. Where the nodes are Documents and Siblings together is called a Collection. Consider the following graph. its the structure of one of Firestore databases.
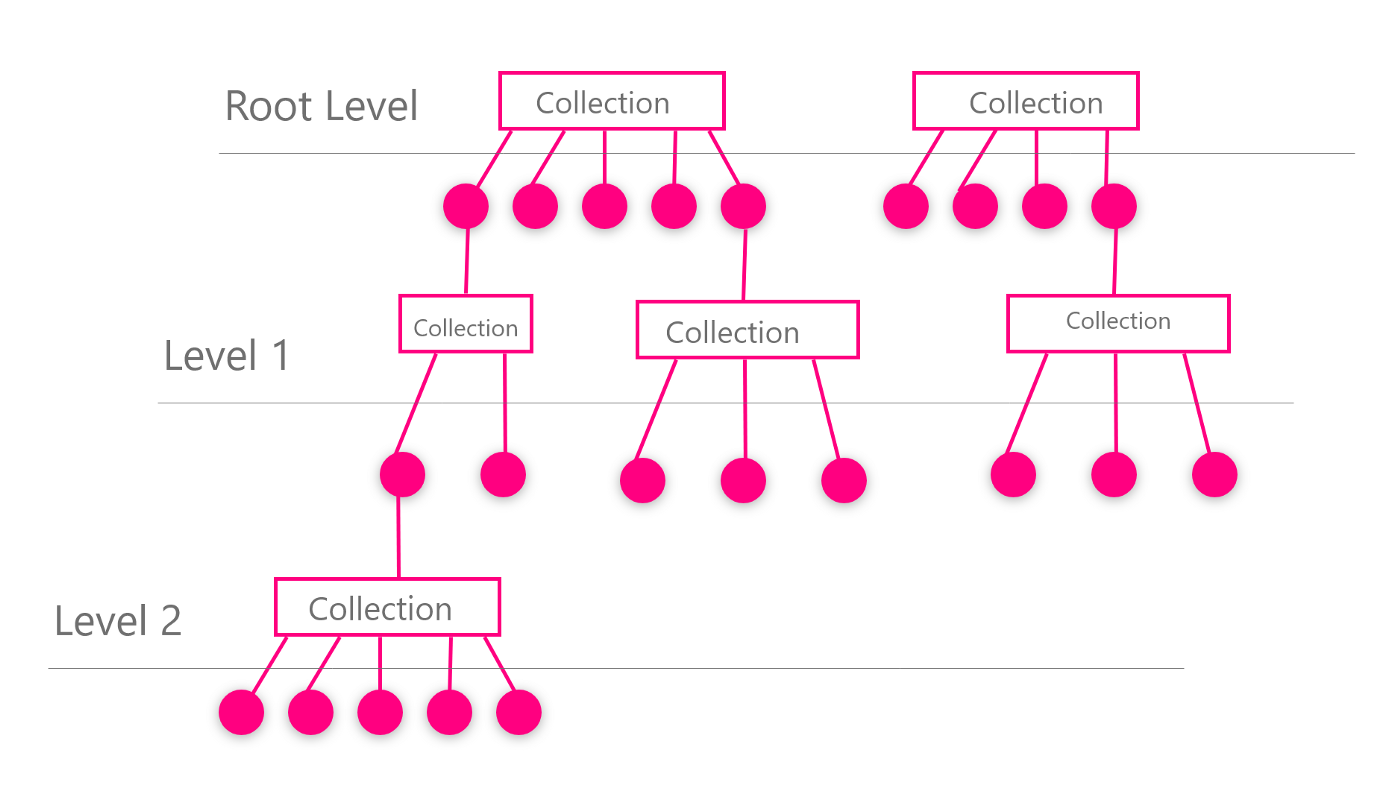
The Circles are the Documents and Rectangle are Collections. this is very important when creating super complex databases with firestore. keeping you database clean is important.
Like all the other traditional databases this architecture has its ups and downs. but at the moment lets try to implement one.
Initialization
First initialized the Firestore above widget build method.
final dbref = Firestore.instance;
this database reference has no collection or document specified. because we are gonna be utilizing a root level collection we wont have to. but if you are using a deeper level database specify its path. (The path have to follow the patter of “Collection — Document — Collection — …” remember to end it in a Collection so otherwise you wont be able to utilize the collection)
Read From The Collection
We can do this in many ways. my favorite one is Using a Stream. but in this tutorial we will do thing in a normal manner. but if you are interested in using a Stream read the following after this tutorial.

First my Database looks like this.
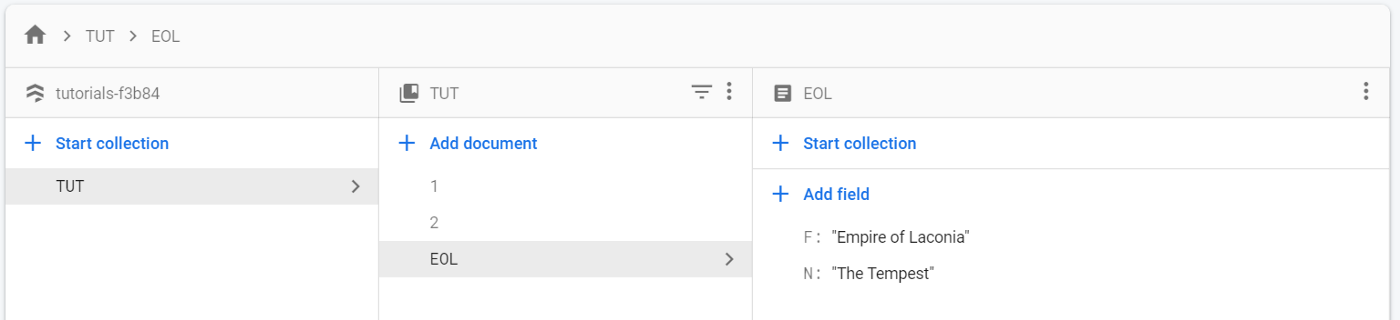
Lets create a Function. it will get data from the Collection.
This will simply get all the documents from the collection ‘TUT’ and print the documents to the console. which looks like this.

The Get-documents will return a List. which you can treat as a normal array.
to get to 0th Document you can go like,
data.documents[0]
to get to a special Field in one particular document lets say “N” according to my DB,
data.documents[0][‘N’]
See? its Easy!.
you can also get some properties of these data objects like documentId. this document id will come in handy in the ‘delete’ section of this tutorial
Add/Update Data
adding data to a Collections is same as to a document. only difference is we provide the collection where it should be a child of.
In this example look at the second function. it save data in,
collection(‘TUT’).document(‘EOLX’).collection(‘SHIPS’)
But in database document ‘EOLX’ does not exists. but this will create that for you and save the data to the document under that ‘Ships’ collection.
Delete
here we get to the Tricky part. reason is we cant delete a collection!.
but we can delete documents in it to make up for that.
what we do is we delete each document. we don’t need to know the name of the document as its provided by “documentID”.
In the next tutorial we will discuss about Building Queries in Firestore.
Note : All the Working State Code for these tutorials can be found in : https://github.com/SrilalS/Flutter-Tutorial