Creating a Real time Data Stream is easy with Flutter Streams and Firestore.
Step 1 : Add Firestore to Your Flutter App
Please read this article to see how to add Firestore to Your Flutter App
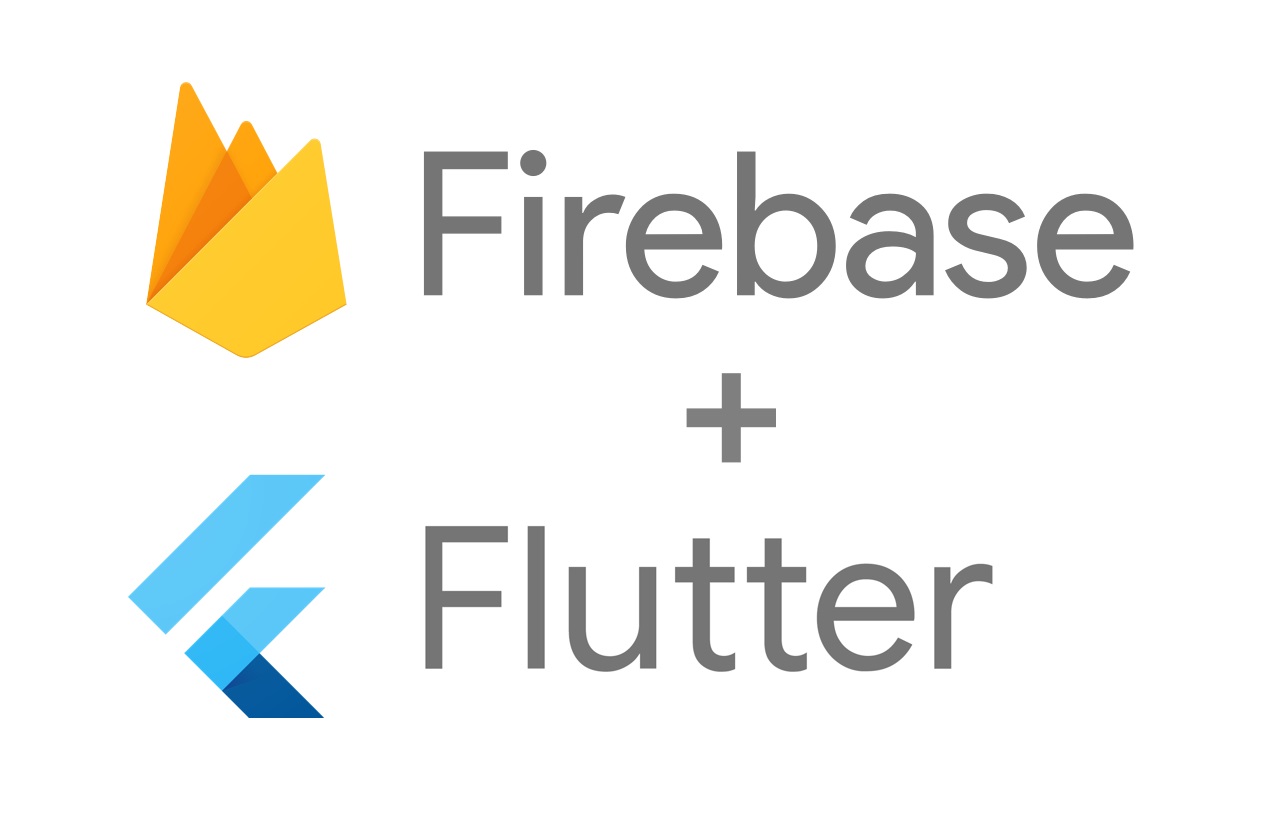
Step 2 : Coding! (yay!)
First create a screen similar to this.
Notice that its important to use either expanded widget or a container widget with defined size because we are going to build a ListView. ListViews Required to be contained in a Sized Container of some sort.
Then add a Stream builder,
Notice the ‘stream’. in here i’m referring to a collection named ‘TUT’ in my database. this will get all the documents inside that database and parse it into a snapshot. we will use this snapshot to get the data we need. in this collection i have 3 documents.
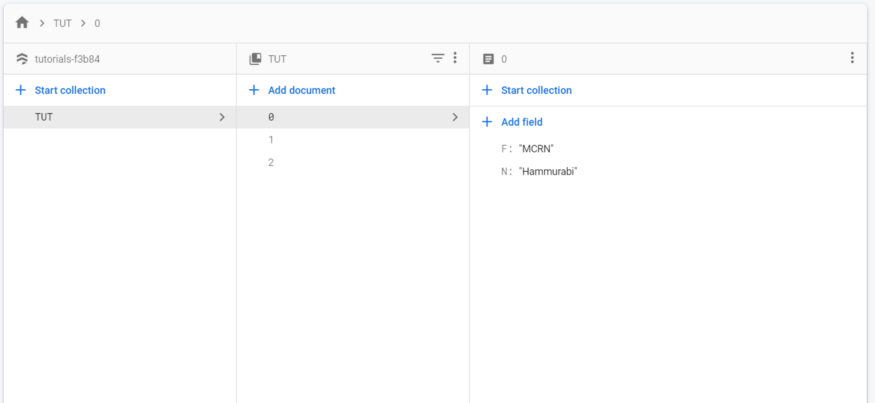
Now create a ListView.Builder() as below. what list view does is it will build one widget per all items in the data snapshot. for each widget it build it will assign a index. please notice that the document name and the index is totally unrelated.
Notice the itemBuilder, inside it we have passed a parameter name index. its will be the unique id for the widgets.
also in the itemCount its important to set a limit. otherwise the list will keep building forever. so we put the limit of how many documents this snapshot has. but you are free to modify it as you want to.
snapshot.data.documents[index][‘N’]
to get to our data we goes in the following path,
Snapshot => Data => Documents =>Document ID using Index => Specific Field of the Document we want
also if the database is empty it will return an empty snapshot. this will result in errors as the ListView needs at-least 1 items. to prevent that add a if condition to check the snapshot to the stream before returning the ListView as below.
Now Run the Project on a internet enabled Phone or a Emulator.
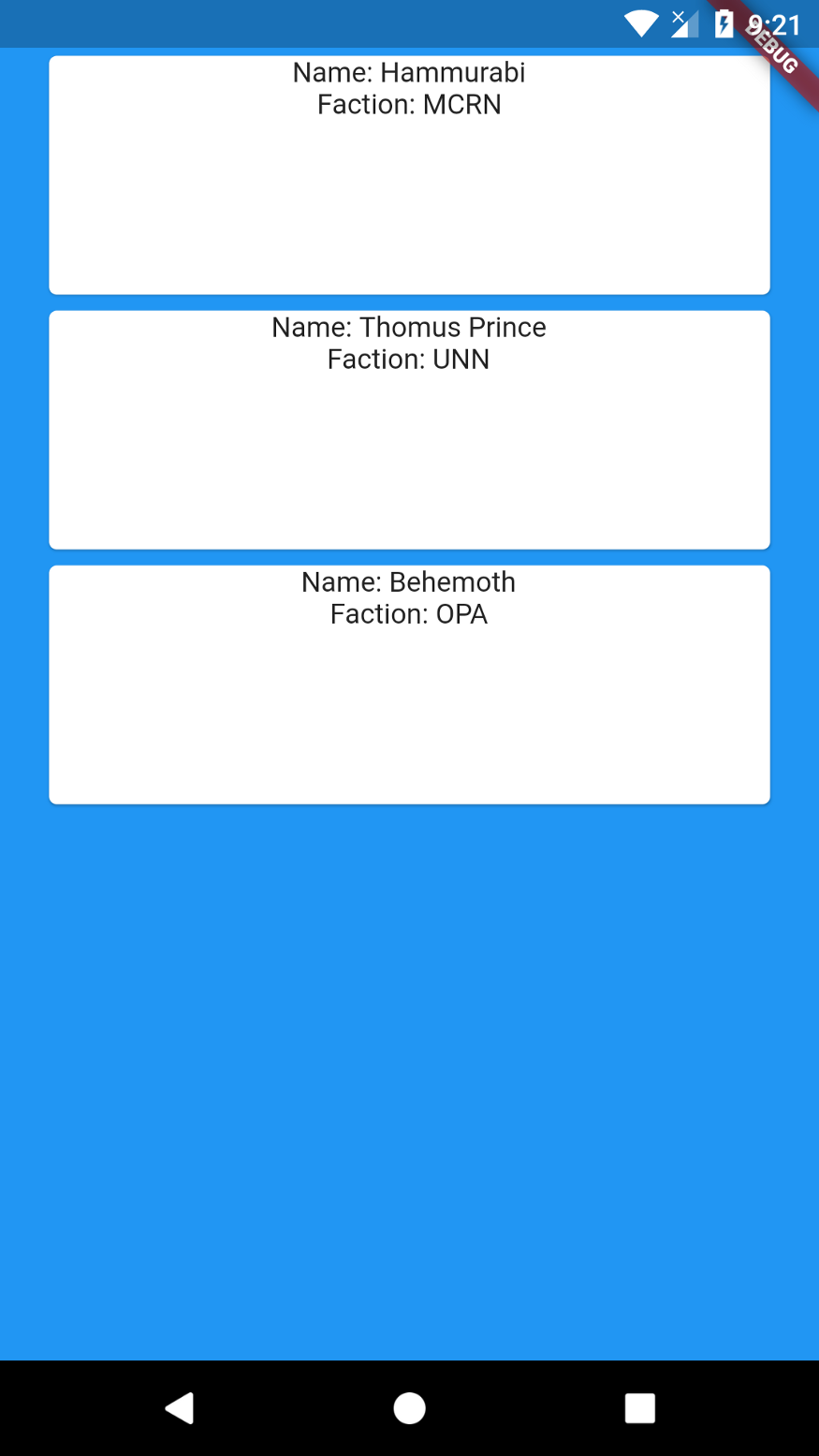
Now, if you added a another Document to the database, it will instantly show up on our list.
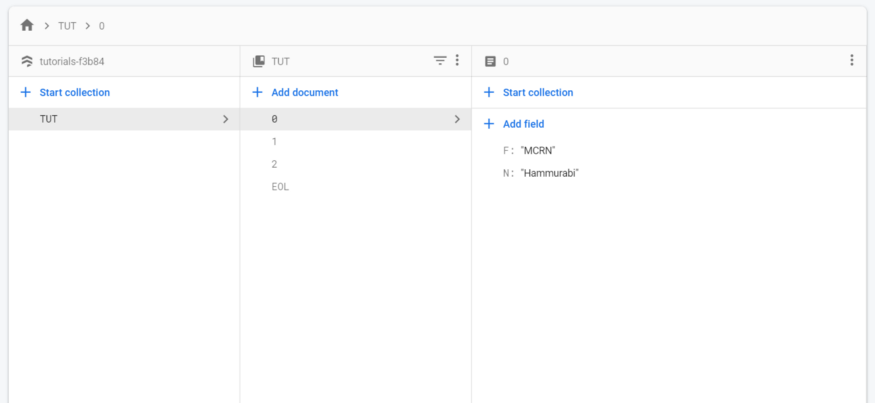
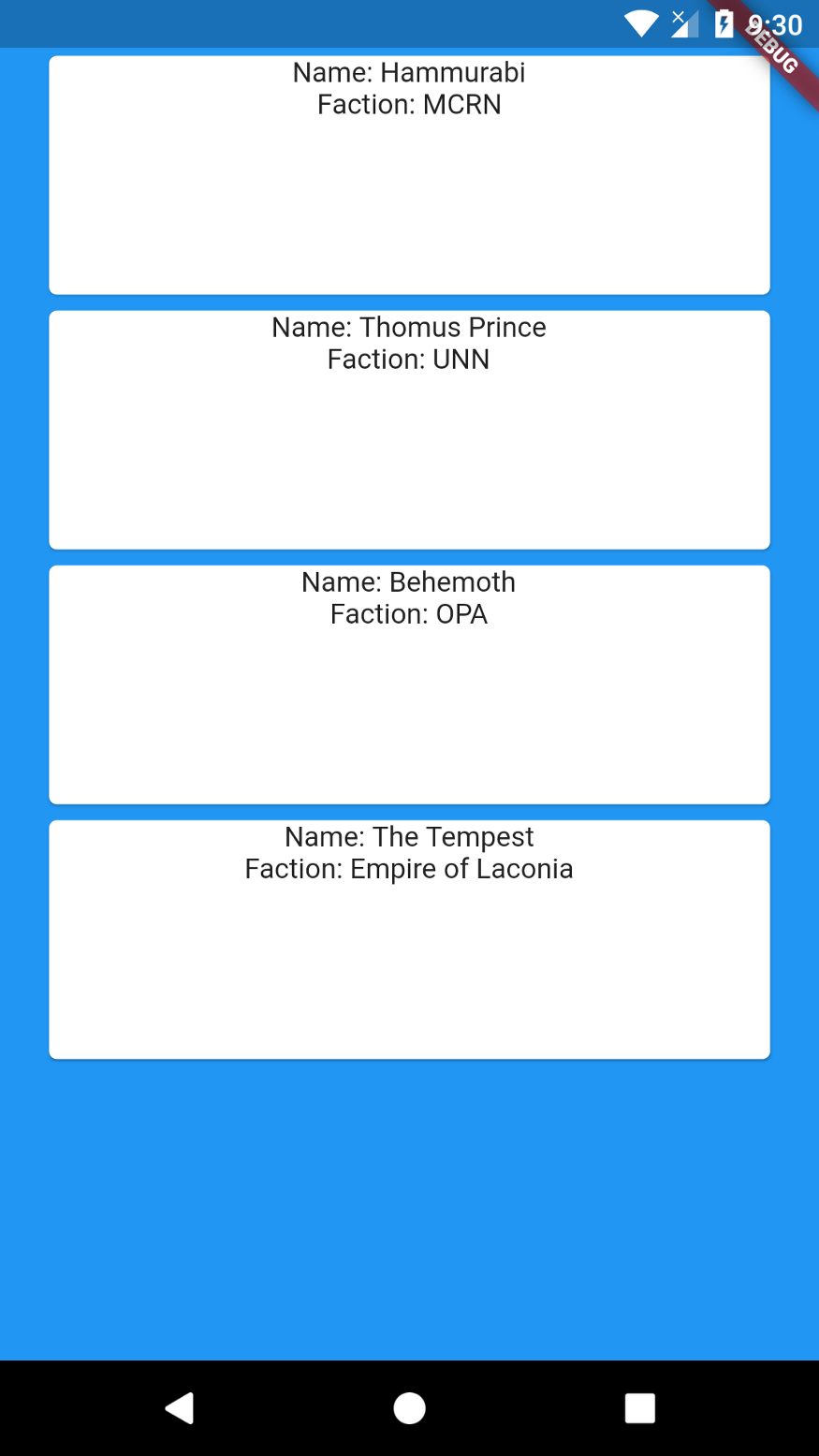
Yay!
Github : https://github.com/SrilalS